HydPy-WQ-Trapeze-Strickler (multi-trapeze river profile submodel including Strickler-based calculations)¶
wq_trapeze_strickler
is a stateless submodel that requires information on the current
water level or depth from its main model (usually a routing model). It returns the
corresponding discharge and related properties, such as the kinematic wave celerity.
See the documentation on the application model musk_mct
, which shows how to use
wq_trapeze_strickler
in practice. Also, please see the documentation on the related
application model wq_trapeze
, which also relies on stacket and symmetric trapezes but
does not include discharge calculations.
wq_trapeze
and wq_trapeze_strickler
offer the same plotting functionalities. To
avoid redundancies, we give a single example for wq_trapeze_strickler
and refer you
to the documentation on wq_trapeze
for further details:
>>> from hydpy.core.testtools import save_autofig
>>> from hydpy.models.wq_trapeze_strickler import *
>>> parameterstep()
>>> nmbtrapezes(3)
>>> bottomlevels(1.0, 3.0, 4.0)
>>> bottomwidths(2.0, 0.0, 2.0)
>>> sideslopes(0.0, 2.0, 2.0)
>>> derived.trapezeheights.update()
>>> derived.slopewidths.update()
>>> figure = model.plot()
>>> save_autofig(f"wq_trapeze_strickler_three_trapezes.png", figure=figure)
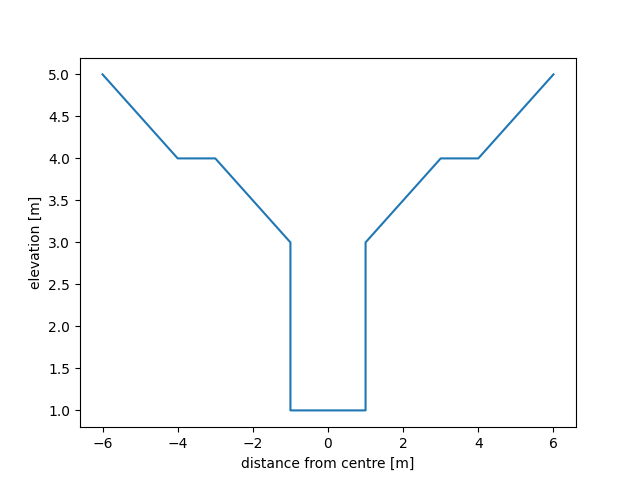
- class hydpy.models.wq_trapeze_strickler.Model[source]¶
Bases:
TrapezeModel
,CrossSectionModel_V1
HydPy-WQ-Trapeze-Strickler (multi-trapeze river profile submodel including Strickler-based calculations).
- The following interface methods are available to main models using the defined model as a submodel:
Use_WaterDepth_V1
Set the water depth in m and use it to calculate all other properties.Use_WaterLevel_V1
Set the water level in m and use it to calculate all other properties.Get_WettedArea_V1
Get the wetted area in m².Get_SurfaceWidth_V1
Get the surface width in m.Get_Discharge_V1
Get the discharge in m³/s.Get_Celerity_V1
Get the wave celerity in m/s.
- The following “additional methods” might be called by one or more of the other methods or are meant to be directly called by the user:
Set_WaterDepth_V1
Set the water depth in m.Set_WaterLevel_V1
Set the water level in m.Calc_WaterDepth_V1
Calculate the water depth based on the current water level.Calc_WaterLevel_V1
Calculate the water level based on the current water depth.Calc_WettedAreas_V1
Calculate the wetted area for each trapeze range.Calc_WettedArea_V1
Sum up the individual trapeze ranges’ wetted areas.Calc_WettedPerimeters_V1
Calculate the wetted perimeter for each trapeze range.Calc_WettedPerimeterDerivatives_V1
Calculate the change in the wetted perimeter of each trapeze range with respect to the water level increase.Calc_SurfaceWidths_V1
Calculate the surface width for each trapeze range.Calc_SurfaceWidth_V1
Sum the individual trapeze ranges’ surface widths.Calc_Discharges_V1
Calculate the discharge for each trapeze range.Calc_Discharge_V2
Sum the individual trapeze ranges’ discharges.Calc_DischargeDerivatives_V1
Calculate the discharge change for each trapeze range with respect to a water level increase.Calc_DischargeDerivative_V1
Sum the individual trapeze ranges’ discharge derivatives.Calc_Celerity_V1
Calculate the kinematic wave celerity.
- DOCNAME: DocName = ('WQ-Trapeze-Strickler', 'multi-trapeze river profile submodel including Strickler-based calculations')¶
- prepare_bottomslope¶
Set the bottom’s slope (in the longitudinal direction) [-].
>>> from hydpy.models.wq_trapeze_strickler import * >>> parameterstep() >>> model.prepare_bottomslope(0.01) >>> bottomslope bottomslope(0.01)
- REUSABLE_METHODS: ClassVar[tuple[type[ReusableMethod], ...]] = ()¶
- cymodel: CyModelProtocol | None¶
- parameters: parametertools.Parameters¶
- sequences: sequencetools.Sequences¶
- masks: masktools.Masks¶
- class hydpy.models.wq_trapeze_strickler.ControlParameters(master: Parameters, cls_fastaccess: type[FastAccessParameter] | None = None, cymodel: CyModelProtocol | None = None)¶
Bases:
SubParameters
Control parameters of model wq_trapeze_strickler.
- The following classes are selected:
NmbTrapezes()
Number of trapezes defining the cross section [-].BottomLevels()
The bottom level for each trapeze [m].BottomWidths()
The bottom width for each trapeze [m].SideSlopes()
The side slope for each trapeze[-].StricklerCoefficients()
Manning-Strickler coefficient for each trapeze [m^(1/3)/s].BottomSlope()
Bottom slope [-].
- class hydpy.models.wq_trapeze_strickler.DerivedParameters(master: Parameters, cls_fastaccess: type[FastAccessParameter] | None = None, cymodel: CyModelProtocol | None = None)¶
Bases:
SubParameters
Derived parameters of model wq_trapeze_strickler.
- The following classes are selected:
BottomDepths()
The cumulated depth of a trapeze and its lower neighbours [m].TrapezeHeights()
The individual height of each trapeze [m].SlopeWidths()
The tatal width of both side slopes of each trapeze.PerimeterDerivatives()
Change of the perimeter of each trapeze relative to a water level increase within the trapeze’s range [-].
- class hydpy.models.wq_trapeze_strickler.FactorSequences(master: Sequences, cls_fastaccess: type[TypeFastAccess_co] | None = None, cymodel: CyModelProtocol | None = None)¶
Bases:
FactorSequences
Factor sequences of model wq_trapeze_strickler.
- The following classes are selected:
WaterDepth()
Water depth [m].WaterLevel()
Water level [m].WettedAreas()
Wetted area of each trapeze range [m²].WettedArea()
Total wetted area [m²].WettedPerimeters()
Wetted perimeter of each trapeze range [m].WettedPerimeterDerivatives()
Change in the wetted perimeter of each trapeze range with respect to a water level increase [-].SurfaceWidths()
Surface width of each trapeze range [m].SurfaceWidth()
Total surface width [m].DischargeDerivatives()
Discharge change of each trapeze range with respect to a water level increase [m²/s].DischargeDerivative()
Total discharge change with respect to a water level increase [m²/s].Celerity()
Kinematic celerity (wave speed) [m/s].
- class hydpy.models.wq_trapeze_strickler.FluxSequences(master: Sequences, cls_fastaccess: type[TypeFastAccess_co] | None = None, cymodel: CyModelProtocol | None = None)¶
Bases:
FluxSequences
Flux sequences of model wq_trapeze_strickler.
- The following classes are selected:
Discharges()
The discharge of each trapeze range [m³/s].Discharge()
Total discharge [m³/s].