HydPy-WQ-Trapeze (multi-trapeze river profile submodel)¶
wq_trapeze
is a stateless “two-way” submodel. It supports calculating properties
like the wetted area for a given water depth and properties like the water depth for a
given wetted area. See the documentation on the application model sw1d_channel
,
which shows the usage of “both ways” in practice. Here, we only aim to visualise how
wq_trapeze
combines multiple symmetric trapezes to a single cross-section profile via
method plot()
.
The following test function helps us to use method plot()
repeatedly and
insert the generated figures into the online documentation:
>>> from matplotlib import pyplot
>>> from hydpy.core.testtools import save_autofig
>>> def plot(example=None, save=True, add_x_y=None, **kwargs):
... derived.trapezeheights.update()
... derived.slopewidths.update()
... figure = model.plot(**kwargs)
... if add_x_y is not None:
... x, y = add_x_y
... pyplot.plot([x, x], [y, 5.0], color=kwargs.get("color"), linestyle=":")
... pyplot.plot([-x, -x], [y, 5.0], color=kwargs.get("color"), linestyle=":")
... if example is not None:
... save_autofig(f"wq_trapeze_{example}.png", figure=figure)
We start with a single trapeze:
>>> from hydpy.models.wq_trapeze import *
>>> parameterstep()
>>> nmbtrapezes(1)
Parameter BottomLevels
does not affect the profile’s shape for a single trapeze. We
set its value to one meter arbitrarily:
>>> bottomlevels(1.0)
With parameter BottomWidths
larger than zero and parameter SideSlopes
being zero,
the single trapeze becomes a simple, infinitely high rectangle:
>>> bottomwidths(2.0)
>>> sideslopes(0.0)
>>> plot("rectangle")
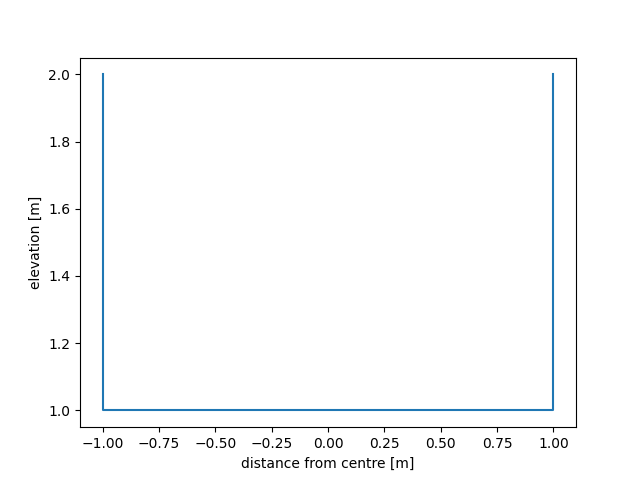
Conversely, the single trapeze becomes a simple, infinitely high triangle:
>>> bottomwidths(0.0)
>>> sideslopes(2.0)
>>> plot("triangle")
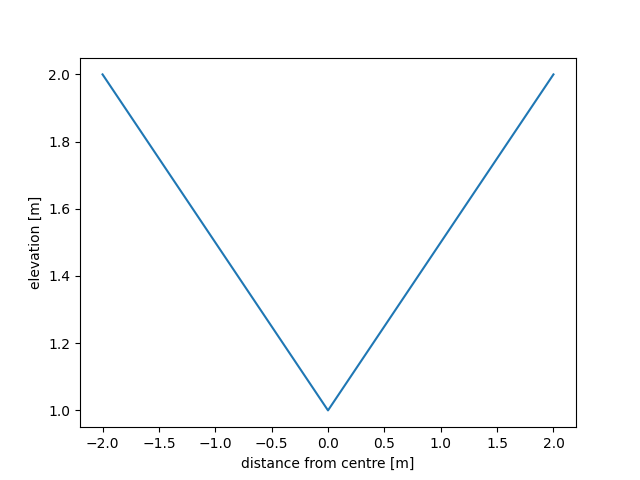
Set both values larger than zero to gain a “complete” trapeze:
>>> bottomwidths(2.0)
>>> sideslopes(2.0)
>>> plot("one_trapeze")
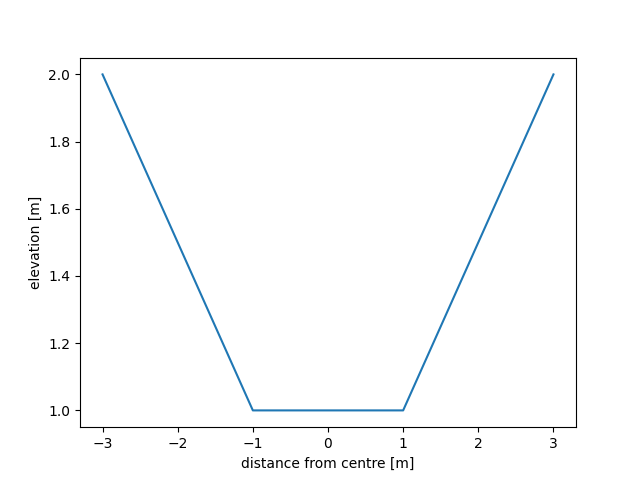
Next, we want to construct a more complex profile consisting of three trapezes:
>>> nmbtrapezes(3)
We must assign them increasing bottom levels (the bottom level of an upper trapeze defines the height of its lower neighbour):
>>> bottomlevels(1.0, 3.0, 4.0)
The following profile combines the three previously defined geometries:
>>> bottomwidths(2.0, 0.0, 2.0)
>>> sideslopes(0.0, 2.0, 2.0)
>>> plot("three_trapezes")
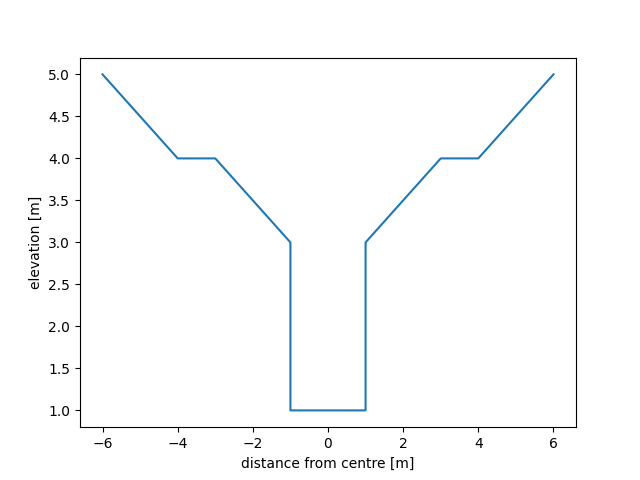
Note that each upper trapeze is stretched according to the width of its lower neighbour. The following example illustrates this more clearly by plotting each trapeze in a different colour:
>>> nmbtrapezes(1)
>>> bottomlevels(1.0)
>>> bottomwidths(2.0)
>>> sideslopes(0.0)
>>> plot(add_x_y=(1.0, 3.0), ymax=3.0, color="green", label="trapeze 1")
>>> bottomlevels(3.0)
>>> bottomwidths(2.0)
>>> sideslopes(2.0)
>>> plot(add_x_y=(3.0, 4.0), ymax=4.0, color="yellow", label="trapeze 2")
>>> bottomlevels(4.0)
>>> bottomwidths(8.0)
>>> sideslopes(2.0)
>>> plot("config_1", ymax=5.0, color="red", label="trapeze 3")
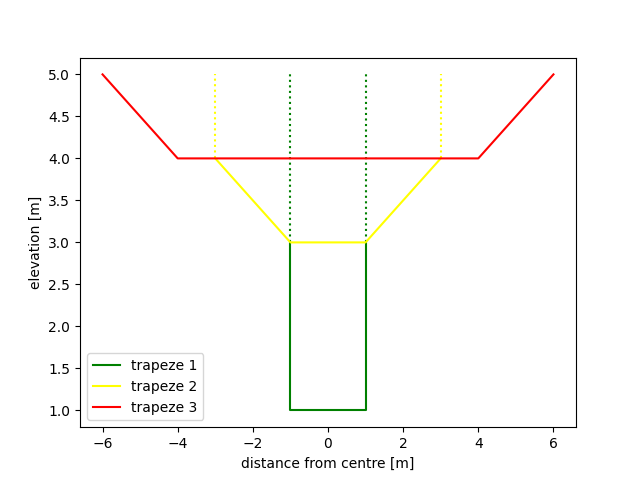
In addition to the configuration options shown in the last example, one can pass True
to the keyword argument label. Then, plot()
tries to include the
responsible element’s name into the legend, which is impossible in this example, so
that “?” serves as a spare:
>>> plot("config_2", ymax=3.0, label=True)
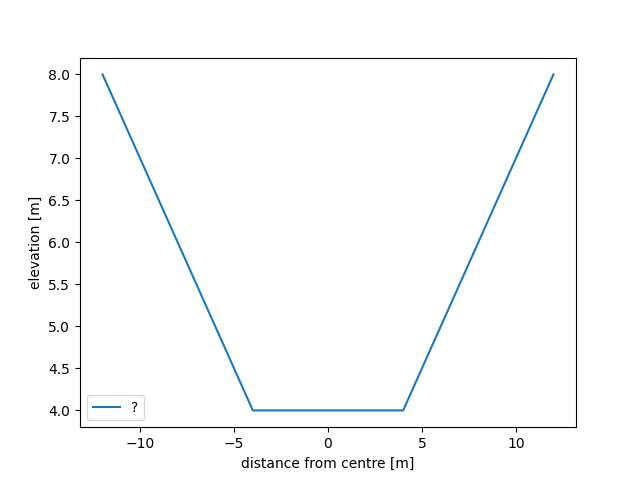
(Upon closer inspection, the last example also shows that plot()
ignores
too low ymax values silently.)
- class hydpy.models.wq_trapeze.Model[source]¶
Bases:
TrapezeModel
,CrossSectionModel_V2
HydPy-WQ-Trapeze (multi-trapeze river profile submodel).
- The following interface methods are available to main models using the defined model as a submodel:
Use_WaterDepth_V2
Set the water depth in m and use it to calculate all other properties.Use_WaterLevel_V2
Set the water level in m and use it to calculate all other properties.Use_WettedArea_V1
Set the wetted area in m² and use it to calculate all other properties.Get_WaterDepth_V1
Get the water depth in m.Get_WaterLevel_V1
Get the water level in m.Get_WettedArea_V1
Get the wetted area in m².Get_WettedPerimeter_V1
Get the wetted perimeter in m.
- The following “additional methods” might be called by one or more of the other methods or are meant to be directly called by the user:
Set_WaterDepth_V1
Set the water depth in m.Set_WaterLevel_V1
Set the water level in m.Set_WettedArea_V1
Set the wetted area in m².Calc_WaterDepth_V1
Calculate the water depth based on the current water level.Calc_WaterDepth_V2
Calculate the water depth based on the current wetted area.Calc_WaterLevel_V1
Calculate the water level based on the current water depth.Calc_WettedAreas_V1
Calculate the wetted area for each trapeze range.Calc_WettedArea_V1
Sum up the individual trapeze ranges’ wetted areas.Calc_WettedPerimeters_V1
Calculate the wetted perimeter for each trapeze range.Calc_WettedPerimeter_V1
Sum up the individual trapeze ranges’ wetted perimeters.Calc_WettedPerimeterDerivatives_V1
Calculate the change in the wetted perimeter of each trapeze range with respect to the water level increase.
- REUSABLE_METHODS: ClassVar[tuple[type[ReusableMethod], ...]] = ()¶
- cymodel: CyModelProtocol | None¶
- parameters: parametertools.Parameters¶
- sequences: sequencetools.Sequences¶
- masks: masktools.Masks¶
- class hydpy.models.wq_trapeze.ControlParameters(master: Parameters, cls_fastaccess: type[FastAccessParameter] | None = None, cymodel: CyModelProtocol | None = None)¶
Bases:
SubParameters
Control parameters of model wq_trapeze.
- The following classes are selected:
NmbTrapezes()
Number of trapezes defining the cross section [-].BottomLevels()
The bottom level for each trapeze [m].BottomWidths()
The bottom width for each trapeze [m].SideSlopes()
The side slope for each trapeze[-].
- class hydpy.models.wq_trapeze.DerivedParameters(master: Parameters, cls_fastaccess: type[FastAccessParameter] | None = None, cymodel: CyModelProtocol | None = None)¶
Bases:
SubParameters
Derived parameters of model wq_trapeze.
- The following classes are selected:
BottomDepths()
The cumulated depth of a trapeze and its lower neighbours [m].TrapezeHeights()
The individual height of each trapeze [m].SlopeWidths()
The tatal width of both side slopes of each trapeze.TrapezeAreas()
The individual area of each trapeze [m].PerimeterDerivatives()
Change of the perimeter of each trapeze relative to a water level increase within the trapeze’s range [-].
- class hydpy.models.wq_trapeze.FactorSequences(master: Sequences, cls_fastaccess: type[TypeFastAccess_co] | None = None, cymodel: CyModelProtocol | None = None)¶
Bases:
FactorSequences
Factor sequences of model wq_trapeze.
- The following classes are selected:
WaterDepth()
Water depth [m].WaterLevel()
Water level [m].WettedAreas()
Wetted area of each trapeze range [m²].WettedArea()
Total wetted area [m²].WettedPerimeters()
Wetted perimeter of each trapeze range [m].WettedPerimeter()
Total wetted perimeter [m].WettedPerimeterDerivatives()
Change in the wetted perimeter of each trapeze range with respect to a water level increase [-].