dam_v007¶
Retention basin version of HydPy-Dam.
dam_v007
is a simple “retention basin” model, similar to the “RUEC”
model of LARSIM. One can understand it as an extension of dam_v006
,
and it partly requires equal specifications. Hence, before continuing
please first read the documentation on dam_v006
.
In extension to dam_v006
, dam_v007
implements the control parameter
AllowedRelease
(and the related parameters WaterLevelMinimumThreshold
and WaterLevelMinimumTolerance
). Usually, one takes the discharge
not causing any harm downstream as the “allowed release”, making dam_v007
behave like a retention basin without active control. However, one can
vary the allowed release seasonally (AllowedRelease
inherits from class
SeasonalParameter
).
In contrast to dam_v006
, dam_v007
does not allow to restrict the speed
of the water level decrease during periods with little inflow and thus does
not use the parameter AllowedWaterLevelDrop
.
Integration tests¶
Note
When new to HydPy, consider reading section How to understand integration tests? first.
We create the same test set as for application model dam_v006
,
including an identical inflow series and an identical relationship
between stage and volume:
>>> from hydpy import IntegrationTest, Element, pub
>>> pub.timegrids = "01.01.2000", "21.01.2000", "1d"
>>> parameterstep("1d")
>>> element = Element("element", inlets="input_", outlets="output")
>>> element.model = model
>>> IntegrationTest.plotting_options.axis1 = fluxes.inflow, fluxes.outflow
>>> IntegrationTest.plotting_options.axis2 = states.watervolume
>>> test = IntegrationTest(element)
>>> test.dateformat = "%d.%m."
>>> test.inits = [(states.watervolume, 0.0)]
>>> watervolume2waterlevel(
... weights_input=1.0, weights_output=1.0,
... intercepts_hidden=0.0, intercepts_output=0.0,
... activation=0)
>>> catchmentarea(86.4)
>>> element.inlets.input_.sequences.sim.series = [
... 0.0, 1.0, 6.0, 12.0, 10.0, 6.0, 3.0, 2.0, 1.0, 0.0,
... 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0]
base scenario¶
To show that dam_v007
extends dam_v006
correctly, we also define the
same quasi-linear relation between discharge and stage used throughout the
integration tests of dam_v006
and additionally set the allowed release to
0 m³/s (which makes the values of the two water-related control parameters
irrelevant). As expected, dam_v007
now calculates outflow values
identical with the ones of the base scenario example of
dam_v006
(where AllowedWaterLevelDrop
is inf
):
>>> waterlevel2flooddischarge(ann(
... weights_input=1.0, weights_output=10.0,
... intercepts_hidden=0.0, intercepts_output=0.0,
... activation=0))
>>> allowedrelease(0.0)
>>> waterlevelminimumtolerance(0.1)
>>> waterlevelminimumthreshold(0.0)
>>> test("dam_v007_base_scenario")
Click to see the table
Click to see the graph
spillway¶
Now, we introduce a more realistic relationship between flood discharge and stage, where the spillway of the retention basin starts to become relevant when the water volume exceeds about 1.4 million m³:
>>> waterlevel2flooddischarge(ann(
... weights_input=10.0, weights_output=50.0,
... intercepts_hidden=-20.0, intercepts_output=0.0))
>>> waterlevel2flooddischarge.plot(0.0, 2.0)
>>> from hydpy.core.testtools import save_autofig
>>> save_autofig("dam_v007_waterlevel2flooddischarge.png")
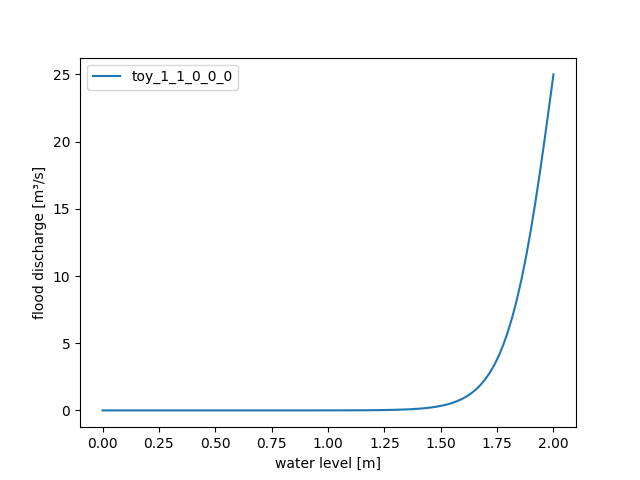
The initially available storage volume of about 1.4 million m³ reduces the peak flow to 7.3 m³/s:
>>> test("dam_v007_spillway")
Click to see the table
Click to see the graph
allowed release¶
In the spillway example, dam_v007
would not handle a
second event following the first one similarly well, due to the retention
basin not releasing the remaining 1.4 million m³ water. Setting the allowed
release to 4 m³/s solves this problem and also decreases the amount of water
stored during the beginning of the event and thus further reduces the peak
flow to 4.6 m³/s:
>>> allowedrelease(4.0)
>>> waterlevelminimumthreshold(0.1)
>>> test("dam_v007_allowed_release")
Click to see the table
Click to see the graph
The initial and final water volumes shown in the last table are slightly
negative, which is due to the periods of zero inflow in combination with
the value of parameter WaterLevelMinimumTolerance
set to 0.1 m. One
could avoid such negative values by increasing parameter
WaterLevelMinimumThreshold
or decreasing parameter
WaterLevelMinimumTolerance
. Theoretically, one could set
WaterLevelMinimumTolerance
to zero, but at the cost of potentially
increased computation times.
-
class
hydpy.models.dam_v007.
Model
[source]¶ Bases:
hydpy.core.modeltools.ELSModel
Version 7 of HydPy-Dam.
- The following “inlet update methods” are called in the given sequence at the beginning of each simulation step:
Pic_Inflow_V1
Update the inlet link sequence.
- The following methods define the relevant components of a system of ODE equations (e.g. direct runoff):
Pic_Inflow_V1
Update the inlet link sequence.Calc_WaterLevel_V1
Determine the water level based on an artificial neural network describing the relationship between water level and water stage.Calc_ActualRelease_V2
Calculate the actual water release in aggrement with the allowed release not causing harm downstream and the actual water volume.Calc_FloodDischarge_V1
Calculate the discharge during and after a flood event based on anSeasonalANN
describing the relationship(s) between discharge and water stage.Calc_Outflow_V1
Calculate the total outflow of the dam.
- The following methods define the complete equations of an ODE system (e.g. change in storage of fast water due to effective precipitation and direct runoff):
Update_WaterVolume_V1
Update the actual water volume.
- The following “outlet update methods” are called in the given sequence at the end of each simulation step:
Pass_Outflow_V1
Update the outlet link sequenceQ
.
-
numconsts
: hydpy.core.modeltools.NumConstsELS¶
-
numvars
: hydpy.core.modeltools.NumVarsELS¶
-
class
hydpy.models.dam_v007.
AideSequences
(master: hydpy.core.sequencetools.Sequences, cls_fastaccess: Optional[Type[FastAccessType]] = None, cymodel: Optional[hydpy.core.typingtools.CyModelProtocol] = None)¶ Bases:
hydpy.core.sequencetools.ModelSequences
[AideSequence
,hydpy.core.variabletools.FastAccess
]Aide sequences of model dam_v007.
- The following classes are selected:
WaterLevel()
Water level [m].
-
class
hydpy.models.dam_v007.
ControlParameters
(master: hydpy.core.parametertools.Parameters, cls_fastaccess: Optional[Type[hydpy.core.parametertools.FastAccessParameter]] = None, cymodel: Optional[hydpy.core.typingtools.CyModelProtocol] = None)¶ Bases:
hydpy.core.variabletools.SubVariables
[hydpy.core.parametertools.Parameters
,Parameter
,hydpy.core.parametertools.FastAccessParameter
]Control parameters of model dam_v007.
- The following classes are selected:
CatchmentArea()
Size of the catchment draining into the dam [km²].WaterLevelMinimumThreshold()
The minimum operating water level of the dam [m].WaterLevelMinimumTolerance()
A tolarance value for the minimum operating water level [m].WaterVolume2WaterLevel()
Artificial neural network describing the relationship between water level and water volume [-].WaterLevel2FloodDischarge()
Artificial neural network describing the relationship between flood discharge and water volume [-].AllowedRelease()
The maximum water release not causing any harm downstream [m³/s].
-
class
hydpy.models.dam_v007.
DerivedParameters
(master: hydpy.core.parametertools.Parameters, cls_fastaccess: Optional[Type[hydpy.core.parametertools.FastAccessParameter]] = None, cymodel: Optional[hydpy.core.typingtools.CyModelProtocol] = None)¶ Bases:
hydpy.core.variabletools.SubVariables
[hydpy.core.parametertools.Parameters
,Parameter
,hydpy.core.parametertools.FastAccessParameter
]Derived parameters of model dam_v007.
- The following classes are selected:
TOY()
References thetimeofyear
index array provided by the instance of classIndexer
available in modulepub
. [-].Seconds()
Length of the actual simulation step size in seconds [s].WaterLevelMinimumSmoothPar()
Smoothing parameter to be derived fromWaterLevelMinimumTolerance
for smoothing kernelsmooth_logistic1()
[m].
-
class
hydpy.models.dam_v007.
FluxSequences
(master: hydpy.core.sequencetools.Sequences, cls_fastaccess: Optional[Type[FastAccessType]] = None, cymodel: Optional[hydpy.core.typingtools.CyModelProtocol] = None)¶ Bases:
hydpy.core.sequencetools.OutputSequences
[FluxSequence
]Flux sequences of model dam_v007.
- The following classes are selected:
Inflow()
Total inflow [m³/s].ActualRelease()
Actual water release thought for reducing drought events downstream [m³/s].FloodDischarge()
Water release associated with flood events [m³/s].Outflow()
Total outflow [m³/s].
-
class
hydpy.models.dam_v007.
InletSequences
(master: hydpy.core.sequencetools.Sequences, cls_fastaccess: Optional[Type[FastAccessType]] = None, cymodel: Optional[hydpy.core.typingtools.CyModelProtocol] = None)¶ Bases:
hydpy.core.sequencetools.LinkSequences
[InletSequence
]Inlet sequences of model dam_v007.
- The following classes are selected:
Q()
Discharge [m³/s].
-
class
hydpy.models.dam_v007.
OutletSequences
(master: hydpy.core.sequencetools.Sequences, cls_fastaccess: Optional[Type[FastAccessType]] = None, cymodel: Optional[hydpy.core.typingtools.CyModelProtocol] = None)¶ Bases:
hydpy.core.sequencetools.LinkSequences
[OutletSequence
]Outlet sequences of model dam_v007.
- The following classes are selected:
Q()
Discharge [m³/s].
-
class
hydpy.models.dam_v007.
SolverParameters
(master: hydpy.core.parametertools.Parameters, cls_fastaccess: Optional[Type[hydpy.core.parametertools.FastAccessParameter]] = None, cymodel: Optional[hydpy.core.typingtools.CyModelProtocol] = None)¶ Bases:
hydpy.core.variabletools.SubVariables
[hydpy.core.parametertools.Parameters
,Parameter
,hydpy.core.parametertools.FastAccessParameter
]Solver parameters of model dam_v007.
- The following classes are selected:
AbsErrorMax()
Absolute numerical error tolerance [m3/s].RelErrorMax()
Relative numerical error tolerance [1/T].RelDTMin()
Smallest relative integration time step size allowed [-].RelDTMax()
Largest relative integration time step size allowed [-].
-
class
hydpy.models.dam_v007.
StateSequences
(master: hydpy.core.sequencetools.Sequences, cls_fastaccess: Optional[Type[FastAccessType]] = None, cymodel: Optional[hydpy.core.typingtools.CyModelProtocol] = None)¶ Bases:
hydpy.core.sequencetools.OutputSequences
[StateSequence
]State sequences of model dam_v007.
- The following classes are selected:
WaterVolume()
Water volume [million m³].